WeChat applet's simple calculator is for your reference. The specific contents are as follows 1. Introduction1. Infix Expression Infix expression is a general method of expressing arithmetic or logical formulas, where operators are placed between operands in infix form. Infix expression is a commonly used arithmetic representation method. Although it is easy for the human brain to understand and analyze infix expressions, they are very complex for computers. Therefore, when calculating the value of an expression, it is usually necessary to convert the infix expression into a prefix or postfix expression before evaluating it. It is very simple for a computer to evaluate a prefix or postfix expression. 2. Postfix Expression Scan the expression from left to right. When a number is encountered, push the number into the stack. When an operator is encountered, pop the two numbers at the top of the stack, use the operator to perform corresponding calculations on them (next top element op + top element of the stack), and push the result into the stack. Repeat the above process until the rightmost end of the expression. The final value obtained by the calculation is the result of the expression. example: (1) 8+4-62 can be expressed as: For example, the postfix expression "3 4 + 5 × 6 -": (1) Scan from left to right and push 3 and 4 into the stack; 2. Program Code1. Code The app.js configuration code is as follows: // app.js App({ onLaunch() { // Display local storage capabilities const logs = wx.getStorageSync('logs') || [] logs.unshift(Date.now()) wx.setStorageSync('logs', logs) // Login wx.login({ success: res => { // Send res.code to the backend in exchange for openId, sessionKey, unionId } }) }, globalData: { userInfo: null }, calculator: express:'', //temporary string strList:[], //infix expression storage (queue first-in, first-out) strListP:[], // suffix expression (queue first-in, first-out) list:[], //Store the stack of operators (first in, last out) calculate:[] //Calculate expression stack (first in, last out) } }) 2. Logic code The code of calculator.js is as follows: // pages/calculator/calculator.js const app = getApp() Page({ /** * Initial data of the page */ data: { operators: ['AC', 'DEL', '%', '/', '7', '8', '9', '×', '4', '5', '6', '+', '1', '2', '3', '-', '0', '.'], res: '=', expression: '0', }, clearAll() { this.setData({ expression: '0', result: '' }) }, click: function (event) { const val = event.target.dataset.value; if (val == 'AC') { this.clearAll(); } else if (val == 'DEL') { if (this.data.expression != '0') { const res = this.data.expression.substr(0, this.data.expression.length - 1); this.setData({ expression: res }) } } else { var len = this.data.expression.length; var s = this.data.expression.substring(len - 1, len); if ((this.checkOperator(s)) && this.checkOperator(val)) { const res = this.data.expression.substr(0, this.data.expression.length); this.setData({ expression: res }) } else { if ((this.data.expression == '0') && (val == '.')) { this.setData({ expression: this.data.expression + String(val) }) } else { this.setData({ expression: this.data.expression === '0' ? val : this.data.expression + String(val) }) } } } }, result() { app.calculator.strList.length = 0; app.calculator.strListP.length = 0; app.calculator.list.length = 0; app.calculator.calculate.length = 0; this.expressToStrList(this.data.expression); let tempList = app.calculator.strList; this.expressToStrListP(tempList); let tempP = app.calculator.strListP for (let m in tempP) { if (this.checkOperator(tempP[m])) { let op1 = app.calculator.calculate[0]; app.calculator.calculate.shift(); let op2 = app.calculator.calculate[0]; app.calculator.calculate.shift(); app.calculator.calculate.unshift(this.countDetail(op2, tempP[m], op1)); } else { app.calculator.calculate.unshift(tempP[m]) } } this.setData({ result: app.calculator.calculate[0] }); }, countDetail(num1, operator, num2) { let result = 0.0; try { if (operator == "×") { result = parseFloat(num1) * parseFloat(num2); } else if (operator == "/") { result = parseFloat(num1) / parseFloat(num2); } else if (operator == "%") { result = parseFloat(num1) % parseFloat(num2); } else if (operator == "+") { result = parseFloat(num1) + parseFloat(num2); } else { result = parseFloat(num1) - parseFloat(num2); } } catch (error) { } return result; }, expressToStrListP(tempList) { //Convert the infix expression set into a postfix expression set for (let item in tempList) { if (this.checkOperator(tempList[item])) { if (app.calculator.list.length == 0) { app.calculator.list.unshift(tempList[item]); } else { if (this.compaerOperator(app.calculator.list[0], tempList[item])) { for (let x in app.calculator.list) { app.calculator.strListP.push(app.calculator.list[x]); } app.calculator.list.length = 0; app.calculator.list.unshift(tempList[item]); } else { app.calculator.list.unshift(tempList[item]); } } } else { app.calculator.strListP.push(tempList[item]); } } if (app.calculator.list.length > 0) { for (let x in app.calculator.list) { app.calculator.strListP.push(app.calculator.list[x]); } app.calculator.list.length = 0; } }, compaerOperator(op1, op2) { if ((op1 == "%" || op1 == "×" || op1 == "/") && (op2 == "-" || op2 == "+")) { return true; } else { return false; } }, expressToStrList(expression) { //Convert string expression into infix queue let temp = ''; for (let i = 0; i < expression.length; i++) { if (i == 0 && expression[i] == "-") { temp = temp + expression[i]; } else { if (this.checkDigit(expression[i])) { temp = temp + expression[i]; } else { if (temp.length > 0) { if (expression[i] == ".") { temp = temp + expression[i]; } else { app.calculator.strList.push(parseFloat(temp)); temp = ''; app.calculator.strList.push(expression[i]); } } else { temp = temp + expression[i]; } } } } if (temp.length > 0 && this.checkDigit(temp.substring(temp.length - 1))) { app.calculator.strList.push(parseFloat(temp)); temp = ''; } }, //Judge whether it is an operator checkOperator(input) { if (input == "-" || input == "+" || input == "/" || input == "%" || input == "×") { return true; } else { return false; } }, // Check if it is a number checkDigit(input) { if ((/^[0-9]*$/.test(input))) { return true; } else { return false; } }, }) 3. Interface code The code of calculator.js is as follows: <!--pages/calculator/calculator.wxml--> <view class="contaniner"> <view class="displayer"> <view class="text">{{expression}}</view> <view class="result">={{result}}</view> </view> <view class="btnArea"> <block wx:for="{{operators}}"> <view class="btn" data-value="{{item}}" capture-bind:tap="click">{{item}}</view> </block> <view class="btn btn1" data-value="{{res}}" bindtap="result">{{res}}</view> </view> </view> 4. Style Code The code of calculator.js is as follows: /* pages/calculator/calculator.wxss */ .container1{ width: 100%; height: 100%; } .displayer{ border: 1px solid #f1f3f3; width: 100%; height: 602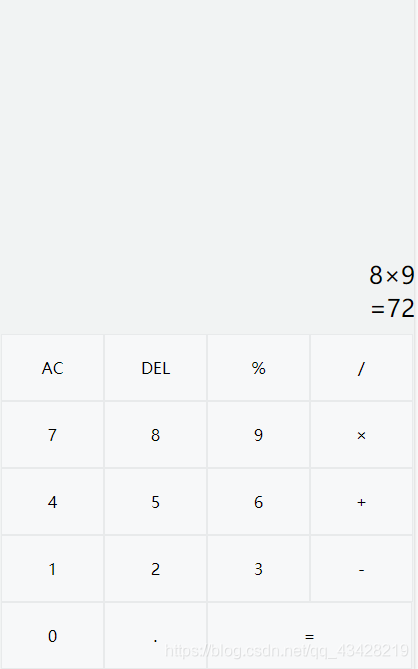 rpx; font-size: 45rpx; background-color: rgba(241, 243, 243, 1.0); } .btnArea{ display: flex; flex-flow: row wrap; justify-content: flex-start; padding: 3rpx; margin: 0; background-color: rgb(241, 243, 243); } .btn{ width: 185rpx; display: flex; align-items: center; height: 120rpx; justify-content: center; border: 1rpx solid #e8eaeb; color: black; background-color: #F7F8F9; } .btn1{ width: 370rpx; } .text{ width: 100%; height: 10%; text-align: right; margin-top: 470rpx; background-color: rgba(241, 243, 243, 1.0); position: absolute; word-break: break-word; } .result{ width: 100%; height: 58rpx; text-align: right; margin-top: 530rpx; background-color: rgba(241, 243, 243, 1.0); position: absolute; word-break: break-word; } 3. Program screenshotsIV. ConclusionUse an array to implement a stack, then convert the expression into an infix expression, and then into a postfix expression, and use the stack to implement the calculation. The above is the full content of this article. I hope it will be helpful for everyone’s study. I also hope that everyone will support 123WORDPRESS.COM. You may also be interested in:
|
<<: Detailed explanation of how to reduce memory usage in MySql
>>: Tips for using the docker inspect command
1. flex-grow, flex-shrink, flex-basis properties ...
Question: In index.html, iframe introduces son.htm...
Table of contents Preface 1. What is a closure? 1...
1. Docker Network Mode When docker run creates a ...
1. Import the module and define a validation stat...
【question】 We have an HP server. When the SSD wri...
Table of contents Related dependency installation...
This article example shares the specific code of ...
One environment Alibaba Cloud Server: CentOS 7.4 ...
The conversion between time, string and timestamp...
Table of contents Message Board Required librarie...
Table of contents What is React Fiber? Why React ...
1. In Windows system, many software installations...
1. The Chinese garbled characters appear in MySQL...
1.vue packaging Here we use the vue native packag...